Add-in Express™ for Microsoft® Office and VSTO
Add-in Express Home > Add-in Express for Office and VSTO > Online Guide > Custom task panes for Outlook and Excel
Custom task panes for Excel and Outlook
To add a new task pane, you add a UserControl to your project and populate it with controls. Then you add an item to the TaskPanes collection of the add-in module and specify its properties:
- Caption - the caption of your task pane (required!)
- Height, Width - the height and width of your task pane (applies to horizontal and vertical task panes, correspondingly)
- DockPosition - you can dock your task pane to the left, top, right, or bottom edges of the host application window
- ControlProgID - the UserControl just added.
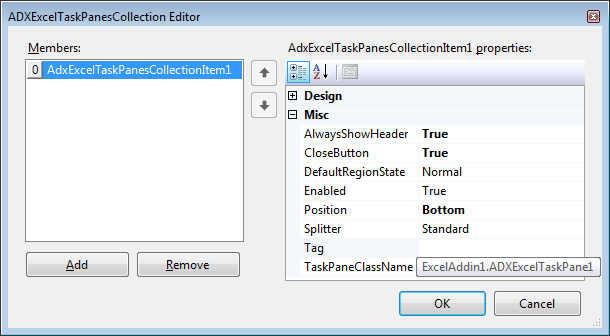
In Add-in Express you work with the task pane component and task pane instances. The TaskPanes collection of the add-in module contains task pane components. When you set, say, the height or dock position of the component, these properties apply to every task pane instance that the host application shows. To modify a property of a task pane instance, you should get the instance itself. This can be done through the Item property of the component (in C#, this property is the indexer for the ADXTaskPane class). The property accepts the Outlook Explorer or Inspector window object that displays the task pane as a parameter. For instance, the method below finds the currently active instance of the task pane in Outlook 2007 and refreshes it. For the task pane to be refreshed in a consistent manner, this method should be called in appropriate event handlers. Private Sub RefreshTaskPane( _
ByVal TaskPaneInstance As _
AddinExpress.VSTO.ADXTaskPane.ADXCustomTaskPaneInstance)
If TaskPaneInstance IsNot Nothing Then
Dim uc As UserControl1 = TaskPaneInstance.Control
If uc IsNot Nothing _
And TaskPaneInstance.Window IsNot Nothing _
Then uc.InfoString = GetSubject(TaskPaneInstance.Window)
End If
End Sub
The InfoString property just gets or sets the text of the Label located on the UserControl1. The GetSubject method is shown below. Private Function GetSubject(ByVal ExplorerOrInspector As Object) As String
Dim mailItem As Outlook.MailItem = Nothing
Dim selection As Outlook.Selection = Nothing
If TypeOf ExplorerOrInspector Is Outlook.Explorer Then
Try
selection = CType(ExplorerOrInspector, Outlook.Explorer).Selection
Catch
End Try
If selection IsNot Nothing Then
If selection.Count > 0 Then mailItem = selection.Item(1)
Marshal.ReleaseComObject(selection)
End If
ElseIf TypeOf ExplorerOrInspector Is Outlook.Inspector Then
mailItem = CType(ExplorerOrInspector, Outlook.Inspector).CurrentItem
End If
If mailItem Is Nothing Then Return ""
Dim subject As String = "The subject is:" + "'" + mailItem.Subject + "'"
If mailItem IsNot Nothing Then Marshal.ReleaseComObject(mailItem)
Return subject
End Function
The code of the GetSubject method emphasizes the following:
- The ExplorerOrInspector parameter was originally obtained through parameters of Add-in Express event handlers. That is why we do not release it (see Releasing COM objects).
- The selection and mailItem variables were obtained "manually" so they must be released.
- All Outlook versions fire an exception when you try to obtain the Selection object in some situations. Below is another sample that demonstrates how the same things can be done in Excel.
Private Sub RefreshTaskPane()
Dim Window As Excel.Window = Me.ExcelApp.ActiveWindow
If Window IsNot Nothing Then
RefreshTaskPane(AdxTaskPane1.Item(Window))
Marshal.ReleaseComObject(Window)
End If
End Sub
Private Sub RefreshTaskPane( _
ByVal TaskPaneInstance As _
AddinExpress.VSTO.ADXTaskPane.ADXCustomTaskPaneInstance)
If TaskPaneInstance IsNot Nothing Then
Dim uc As UserControl1 = TaskPaneInstance.Control
If uc IsNot Nothing And _
TaskPaneInstance.Window IsNot Nothing _
Then
Dim ActiveCell As Excel.Range = _
CType(TaskPaneInstance.Window, Excel.Window).ActiveCell
If ActiveCell IsNot Nothing Then
'relative address
Dim Address As String = _
ActiveCell.AddressLocal(False, False)
Marshal.ReleaseComObject(ActiveCell)
uc.InfoString = "The current cell is " + Address
End If
End If
End If
End Sub
The InfoString property mentioned above just updates the text of the label located on the UserControl. Please pay attention to releasing COM objects in this code.
More about task panes and other features for Excel and Outlook |