Add-in Express™ for Internet Explorer® and Microsoft® .net
Add-in Express Home > Add-in Express for Internet Explorer > Online Guide > Intercepting IE and HTML events, keyboard shortcuts
IE events, HTML events, keyboard shortcuts
Internet Explorer add-on, IE toolbar and IE bar projects created with Add-in Express for IE and .NET support intercepting three event types: IE events, HTML events and keyboard shortcuts.
The sections below provide useful code samples demonstrating what you can do in these events:
Intercepting IE events
IE provides a number of events such as DownloadComplete or BeforeNavigate2. All such events are mapped to the corresponding events of the IEModule class.
The following code samples demonstrate a possible use of such events:
VB.NET
Redirecting to a web site:
Imports AddinExpress.IE
Imports IE = Interop.SHDocVw
'...
Private Sub IEModule_BeforeNavigate2 _
(e As ADXIEBeforeNavigate2EventArgs) Handles MyBase.BeforeNavigate2
If e.Url.ToString().ToLower().Contains("www.site1.com") Then
e.Cancel = True
Me.IEApp.Navigate2("www.site2.com")
End If
End Sub
VB.NET
Modifying the document by adding a script and a button that calls the script:
Private Sub IEBarModule_DownloadComplete() Handles MyBase.DownloadComplete
If ThisIsMyPage(Me.HTMLDocument.url) Then
Dim doc As mshtml.HTMLDocument = Me.HTMLDocument
If doc.body IsNot Nothing Then
Try
Dim myScript As String = _
"function ShowAlert(){alert(""" & "Hello" & """);}"
Dim isMyScriptCreated As Boolean = False
Dim scripts As mshtml.IHTMLElementCollection = doc.scripts
If scripts IsNot Nothing Then
For i As Integer = 0 To scripts.length - 1
Dim script As mshtml.IHTMLScriptElement = _
TryCast(scripts.item(Nothing, i), _
mshtml.IHTMLScriptElement)
If script IsNot Nothing Then
If script.text IsNot Nothing Then
If script.text = myScript Then
isMyScriptCreated = True
End If
End If
Marshal.ReleaseComObject(script)
End If
If isMyScriptCreated Then Exit For
Next
Marshal.ReleaseComObject(scripts)
End If
If Not isMyScriptCreated Then
Dim head As mshtml.HTMLHeadElementClass = _
TryCast(CType(doc.all.tags("head"), _
mshtml.IHTMLElementCollection).item(Nothing, 0), _
mshtml.HTMLHeadElementClass)
If head IsNot Nothing Then
Dim script As mshtml.IHTMLScriptElement = _
CType(doc.createElement("script"), _
mshtml.IHTMLScriptElement)
script.type = "text/javascript"
script.text = myScript
head.appendChild(script)
End If
End If
Catch e As InvalidCastException
System.Windows.Forms.MessageBox.Show( _
New MyWinApiWindow(Me.ParentHandle), _
e.Message + vbCrLf + e.StackTrace)
End Try
Dim newButton As String = _
"<input type=""button"" name=""myButton""" + _
onClick=""ShowAlert();"" value=""Click me!"">"
Me.HTMLDocument.body.insertAdjacentHTML("beforeEnd", newButton)
End If
End If
End Sub
VB.NET
Module-script two-way interaction.
Imports System.Windows.Forms
Imports AddinExpress.IE
Imports System.Reflection
'...
Private Sub IEToolbar_DownloadComplete() Handles MyBase.DownloadComplete
If Not ThisIsMyPage(Me.HTMLDocument.url) Then Return
Try
Dim scriptEngine As Object = Me.HTMLDocument.Script
scriptEngine.GetType().InvokeMember("myAddon",_
BindingFlags.SetProperty, Nothing, scriptEngine, New Object() {Me})
Catch ex As Exception
End Try
'<html><head><script type="text/javascript">
'var myAddon = null;
'function MyFunction()
'{
' if(myAddon != null)
' {
' myAddon.MyMethod("okay");
' }
' else
' alert("The add-on isn't registered");
'}
'</script></head><body>
'<input type="button" name="button1" value="Click me!" onclick="MyFunction();">
'</body></html>
End Sub
Public Sub MyMethod(param As String)
MessageBox.Show(New MyWinApiWindow(Me.ParentHandle), param)
End Sub
See related how-to video:
Intercepting HTML events
Right-click the designer surface of the IE module and choose Add HTML Events in the context menu.
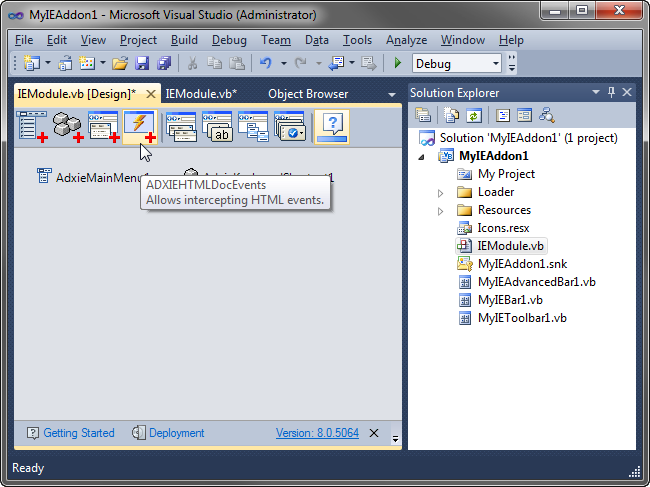
This adds an ADXIEHTMLDocEvents component onto the module.
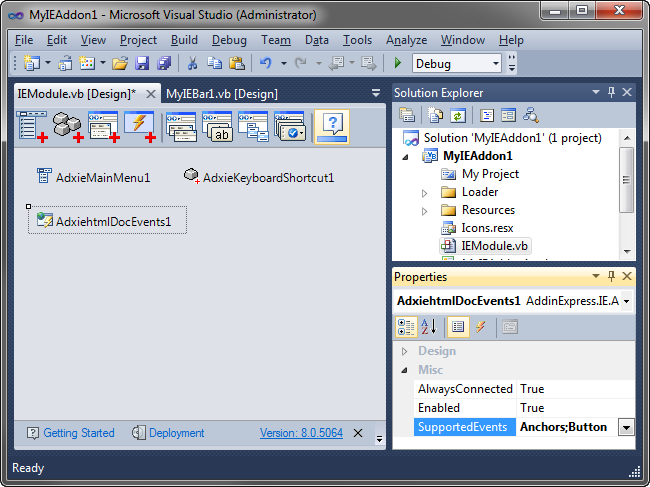
In the SupportedEvents property of the component, select HTML control type(s) the events of which the component will intercept.
Then add event handlers to appropriate events of the component. Every such event (OnClick is an example) provides the eventObject parameter.
See how we use it in the code below:
VB.NET
Intercepting clicking on HTML anchor elements.
Private Sub AdxiehtmlDocEvents1_OnClick( _
ByVal sender As System.Object, _
ByVal eventObject As System.Object, _
ByVal e As _
AddinExpress.IE.ADXCancelEventArgs) _
Handles AdxiehtmlDocEvents1.OnClick
'System.Diagnostics.Debugger.Launch()
Dim eventObj As mshtml.IHTMLEventObj2 = _
CType(eventObject, mshtml.IHTMLEventObj2)
Dim elem As mshtml.IHTMLElement = _
Me.HTMLDocument.elementFromPoint(eventObj.clientX, eventObj.clientY)
If elem IsNot Nothing Then
If TypeOf elem Is mshtml.IHTMLAnchorElement Then
Dim anchor As mshtml.IHTMLAnchorElement = _
CType(elem, mshtml.IHTMLAnchorElement)
Dim window As MyWinApiWindow = New MyWinApiWindow(Me.ParentHandle)
If System.Windows.Forms.MessageBox.Show(window, _
"You've clicked the link labelled '" _
+ elem.innerText _
+ "' and pointing to a page at " _
+ anchor.hostname + "." + vbCrLf + vbCrLf + _
"Do you want to continue?", _
Me.ModuleName, MessageBoxButtons.YesNo) _
= MsgBoxResult.No _
Then
e.Cancel = True
End If
End If
End If
End Sub
The MyWinApiWindow type above is a simple class implementing the System.Windows.Forms.IWin32Window interface (see MyWinApiWindow Class).
The window handle for MyWindow is supplied by the ParentHandle property of the module that returns the handle (hwnd) of the tab window.
Note that you must show messages in this way; otherwise your message box may be shown behind the IE window.
Intercepting keyboard shortcuts
Right-click the designer surface of the IE module and choose Add Keyboard Shortcut in the context menu.
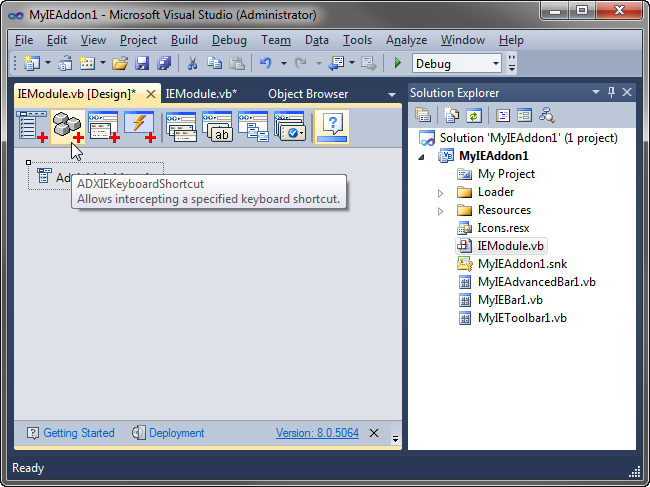
This adds an ADXIEKeyboardShortcut component onto the module. Specify the keyboard shortcut in the ADXIEKeyboardShortcut.ShortcutText property
of the component and handle the OnAction event. Note however that intercepting "native" Internet Explorer shortcuts is not possible. Also note that using the
component requires setting the HandleShortcuts property of the module to true.
VB.NET
Executing a script.
Private Sub AdxieKeyboardShortcut1_OnAction(sender As System.Object) _
Handles AdxieKeyboardShortcut1.OnAction
Me.HTMLDocument.parentWindow.execScript("Alert()", Nothing)
End Sub
|