Ribbon Designer for Microsoft® SharePoint® and and Office 365
Add-in Express Home > Ribbon Designer for SharePoint and Office 365 > Online Guide > Ribbon Designer programmability
Ribbon Designer programmability
Localization
The Ribbon Designer supports localization by providing the Language and Localizable properties: click the designer surface
and look at the Properties window. See also
Localizing SharePoint solutions.
Accessing ribbon controls in JavaScript
If you have a text box with its ID set to "AdxspRibbonTextBox1", then you can write the code below in the OnClick event of a Ribbon button:
SPRibbonProject.SPRibbon.prototype.AdxspRibbonButton2OnClick_EventHandler =
function (sender) {
var textBox = this.FindControl("AdxspRibbonTextBox1");
var isOk = false;
if (textBox != null) {
var text = textBox.Text;
if (text != null && text != "") {
isOk = true;
alert(text);
}
}
if (!isOk) alert("Something went wrong or no text was supplied");
sender.AllowPostBack = false;
}
That is, in the event handler above, you find the text box using the FindControl method; it accepts the ID of a control to find.
Then the text is extracted and an alert is shown.
When testing the method above, you'll find that FindControl() returns the previous value of the text box unless you press
{Enter} when entering text. Alas, this is how the Ribbon text box works and you can do nothing about this.
Creating ribbon controls dynamically
For Ribbon controls containing a menu (e.g. ADXSPRibbonSplitButton), you use the property PopulateDynamically
to populate the menu dynamically. Setting this property to True generats the OnMenuCreate event on the
corresponding JavaScript object. You handle this event to create Ribbon controls on the client side.
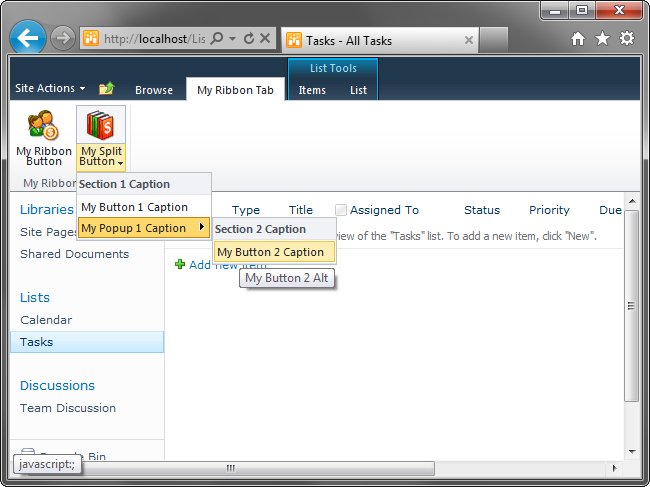
In the screenshot above, the split button is created at design time as described in
Creating a custom SharePoint ribbon tab.
The controls residing on the menu part of the split button are created with this code:
SPRibbonProject.SPRibbon.prototype.CreateMenu = function (sender) {
// Section 1
var menuSection = sender.AddMenuSection("MySection1ID");
menuSection.Caption = "Section 1 Caption";
// menuSection.DisplayMode = "Image16AndCaption";
// Button 1
var button = menuSection.AddControl("MyButton1ID", "Button");
button.Description = "My Button 1 Description.";
button.Caption = "My Button 1 Caption";
// button.Alt = "My Button 1 Alt.";
// button.Enabled = true;
// button.ToolTipTitle = "My Button 1 Tooltip Title";
// button.ToolTipDescription = "My Button 1 Tooltip Description";
// button.ToolTipHelpKeyWord = "My Button 1";
// button.ToolTipShortcutKey = "Ctrl+Shift+Z";
button.OnClickEventName = "ShowId";
var popup = menuSection.AddControl("MyPopup1ID", "FlyoutAnchor");
popup.PopulateDynamically = false;
popup.Caption = "My Popup 1 Caption";
// popup.OnMenuCreateEventName = "OnPopulate_DynamicHandler";
var menuSection2 = popup.AddMenuSection("MySection2ID ");
menuSection2.Caption = "Section 2 Caption";
var button2 = menuSection2.AddControl("MyButton2ID", "Button");
button2.Description = "My Button 2 Description.";
button2.Caption = "My Button 2 Caption";
button2.Alt = "My Button 2 Alt";
button2.OnClickEventName = "ShowId";
}
SPRibbonProject.SPRibbon.prototype.ShowId = function (sender) {
alert(sender.getId());
}
Preserving the state of ribbon controls
All components representing Ribbon controls allow retaining the controls' state. Both ADXSPRibbon and ADXSPPageRibbon
classes have the AutoSaveMode property that you can set to Local, Session (default) or None. All Ribbon components
provide the AutoSaveState property accepting True (default) or False. You can specify whether to preserve the state
of a given Ribbon control at runtime: just set the AutoSave property to False in the JavaScript event OnBeforeSaveState
that every Ribbon component provides.
Ribbon Designer events
The ADXSPRibbon class provides events listed below.
Event name
|
Event description
|
OnAction
|
Occurs when the designer class is invoked via the Submit method of the Ribbon Designer client
object model. Allows handling custom actions and passing data to them. A sample of use is given in
Client-side events. Not supported in sandboxed solutions.
|
OnBeforeRibbonLoad
|
Occurs before the Ribbon XML is supplied to the Server Ribbon. Allows customizing the Ribbon XML
at run-time. Not supported in sandboxed solutions.
|
OnBeforeScriptLoad
|
Occurs before the Ribbon Designer client library is registered for the Server Ribbon.
Allows customizing the Ribbon Designer script at run-time. Not supported in sandboxed solutions.
|
OnDeploymentAction
|
Occurs when the designer feature is installed, uninstalled, activated, deactivated, or upgraded.
|
OnInitialize
|
JavaScript. Occurs when the Ribbon Designer client library is loaded by the browser.
You can use this event to initialize the state of Ribbon controls. A sample of use is given in
Server-side events.
|
OnLoad
|
Occurs when the Ribbon Designer is loaded into the Page object. Not supported in sandboxed solutions.
|
OnRibbonReady
|
JavaScript. Occurs when the ribbon control is constructed. You can use this event to activate a Ribbon
tab (SelectTabById) or check if a tab is available (IsTabAvailable).
|
OnScriptLoaded
|
JavaScript. Occurs when the Ribbon Designer script is loaded into the Page. You can use this event to
perform actions depending on whether your script is loaded.
|
OnSubmit
|
JavaScript. Occurs when the FORM is about to be submitted.
|
|